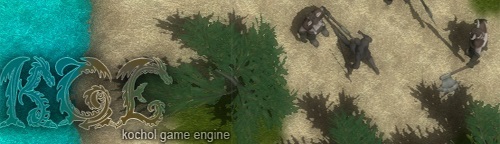 |
Kochol Game Engine
0.1.0
|
Go to the documentation of this file.
10 #include "../kgedef.h"
12 #include "../sn/Sound3D.h"
13 #include "../sn/Sound2D.h"
38 virtual bool Init(
void* hwnd) = 0;
71 virtual void Pause(
bool pause) = 0;
74 virtual void Stop() = 0;
92 #endif // SOUND_SYSTEM_H