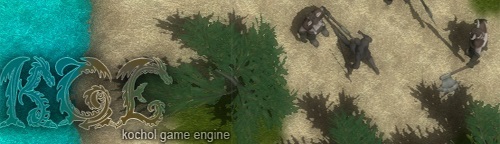 |
Kochol Game Engine
0.1.0
|
Go to the documentation of this file.
9 #include "../math/Matrix.h"
10 #include "../math/Vector.h"
144 float f = (float)sqrt(
X*
X +
Y*
Y +
Z*
Z);
165 X=v1.
Y*v2.
Z-v1.
Z*v2.
Y;
166 Y=v1.
Z*v2.
X-v1.
X*v2.
Z;
167 Z=v1.
X*v2.
Y-v1.
Y*v2.
X;
294 # pragma pack(push, packing)
297 #elif defined(__GNUC__)
298 # define PACK_STRUCT __attribute__((packed))
300 # error compiler not supported
330 # pragma pack(pop, packing)
339 #endif // GFX_STRUCTS_H