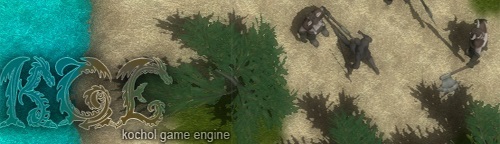 |
Kochol Game Engine
0.1.0
|
Go to the documentation of this file.
9 #include "../Resource.h"
32 Image(
const u32 Handle,
const char* FileName,
const char* Name,
void* ExtraParam);
35 Image(
const char* Name);
41 bool CreateImage(
int Width,
int Height,
int Depth = 1,
int BytesPerPixel = 4,
45 u8* GetData(
u32 MipMapLevel = 0);
48 u32 GetPixels(
int XOff,
int YOff,
int ZOff,
int Width,
int Height,
int Depth,
52 u32 SetData(
u8* pData);
55 void SetPixels(
int XOff,
int YOff,
int ZOff,
int Width,
int Height,
int Depth,
65 bool BuildTileMipMap(
int NumCols,
int NumRows);
89 bool LoadImageFromFile();
92 void CheckDevilErrors(
const char* TextureName );
100 #endif // KGE_GFXIMAGE_H